Note
Go to the end to download the full example code
Use the CRM corpus¶
This shows how to use the CRM corpus functions.
@author: rkmaddox
from expyfun._utils import _TempDir
from expyfun import ExperimentController, analyze, building_doc
from expyfun.stimuli import (crm_prepare_corpus, crm_sentence, crm_info,
crm_response_menu, add_pad, CRMPreload)
import numpy as np
print(__doc__)
crm_path = _TempDir()
fs = 40000
Prepare the corpus¶
For simplicity, here we prepare just two talkers at the native 40000 Hz sampling rate.
Note
For your experiment, you only need to prepare the corpus once per sampling rate, you should probably use the default path, and you should just do all the talkers at once. For the example, we are using fs=40000 and only doing two talkers so that the stimulus preparation is very fast, and a temp directory so that we don’t interfere with any other prepared corpuses. Your code will likely look like this line, and not appear in your actual experiment script:
>>> crm_prepare_corpus(24414)
crm_prepare_corpus(fs, path_out=crm_path, overwrite=True,
talker_list=[dict(sex=0, talker_num=0),
dict(sex=1, talker_num=0)])
# print the valid callsigns
print('Valid callsigns are {0}'.format(crm_info()['callsign']))
# read a sentence in from the hard drive
x1 = 0.5 * crm_sentence(fs, 'm', '0', 'c', 'r', '5', path=crm_path)
# preload all the talkers and get a second sentence from memory
crm = CRMPreload(fs, path=crm_path)
x2 = crm.sentence('f', '0', 'ringo', 'green', '6')
x = add_pad([x1, x2], alignment='start')
Preparing sex 0.
Preparing talker 0.
Preparing sex 1.
Preparing talker 0.
Finished in 0.0 minutes.
Valid callsigns are ['charlie', 'ringo', 'laker', 'hopper', 'arrow', 'tiger', 'eagle', 'baron']
Now we actually run the experiment.
max_wait = 0.01 if building_doc else 3
with ExperimentController(
exp_name='CRM corpus example', window_size=(720, 480),
full_screen=False, participant='foo', session='foo', version='dev',
output_dir=None, stim_fs=40000) as ec:
ec.screen_text('Report the color and number spoken by the female '
'talker.', wrap=True)
screenshot = ec.screenshot()
ec.flip()
ec.wait_secs(max_wait)
ec.load_buffer(x)
ec.identify_trial(ec_id='', ttl_id=[])
ec.start_stimulus()
ec.wait_secs(x.shape[-1] / float(fs))
resp = crm_response_menu(ec, max_wait=0.01 if building_doc else np.inf)
if resp == ('g', '6'):
ec.screen_prompt('Correct!', max_wait=max_wait)
else:
ec.screen_prompt('Incorrect.', max_wait=max_wait)
ec.trial_ok()
analyze.plot_screen(screenshot)
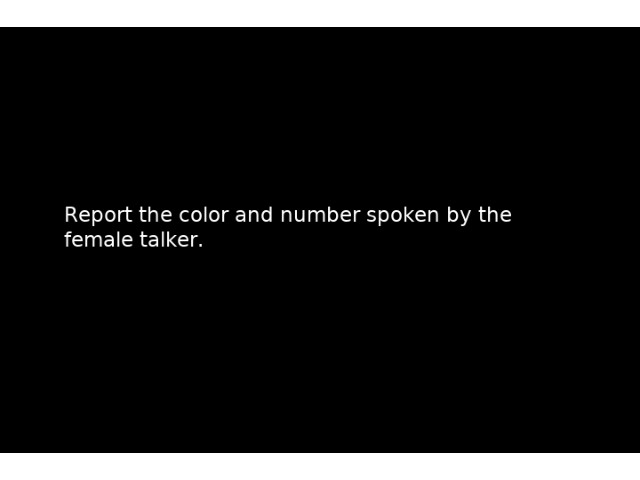
exp_name: CRM corpus example
date: 2023-07-25 19_08_44.189682
file: /home/circleci/project/examples/stimuli/crm_stimuli.py
participant: foo
session: foo
2023-07-25 19:08:44,189 - INFO - Expyfun: Using version 2.0.0.dev0 (requested dev)
2023-07-25 19:08:44,190 - INFO - Expyfun: Setting up sound card using pyglet backend with 2 playback channels
2023-07-25 19:08:44,360 - WARNING - Expyfun: Mismatch between reported stim sample rate (40000) and device sample rate (44100.0). Experiment Controller will resample for you, but this takes a non-trivial amount of processing time and may compromise your experimental timing and/or cause artifacts.
2023-07-25 19:08:44,360 - INFO - Expyfun: Setting up screen
2023-07-25 19:08:44,390 - EXP - Expyfun: Set screen visibility True
2023-07-25 19:08:44,398 - INFO - Initialized [720 480] window on screen XlibScreen(display=<pyglet.canvas.xlib.XlibDisplay object at 0x7f1a95b502b0>, x=0, y=0, width=1400, height=900, xinerama=0) with DPI 69.73
2023-07-25 19:08:44,398 - INFO - Expyfun: Initializing dummy triggering mode
2023-07-25 19:08:44,399 - INFO - Expyfun: Initialization complete
2023-07-25 19:08:44,399 - EXP - Expyfun: Participant: foo
2023-07-25 19:08:44,399 - EXP - Expyfun: Session: foo
2023-07-25 19:08:44,439 - WARNING - Expyfun: Resampling 2.01 seconds of audio
2023-07-25 19:08:44,479 - WARNING - Expyfun: Stimulus max RMS (0.025984056293964386) exceeds stated RMS (0.01) by more than 6 dB.
/home/circleci/project/expyfun/_experiment_controller.py:1849: UserWarning: Expyfun: Stimulus max RMS (0.025984056293964386) exceeds stated RMS (0.01) by more than 6 dB.
warnings.warn(warn_string)
2023-07-25 19:08:44,480 - EXP - Expyfun: Loading 177652 samples to buffer
2023-07-25 19:08:44,481 - EXP - Expyfun: Stamp trial ID to ec_id :
2023-07-25 19:08:44,481 - EXP - Expyfun: Stamp trial ID to ttl_id : []
2023-07-25 19:08:44,481 - EXP - Stamping TTL triggers: []
2023-07-25 19:08:44,481 - EXP - Expyfun: Starting stimuli: flipping screen and playing audio
2023-07-25 19:08:44,483 - EXP - Stamping TTL triggers: [1]
2023-07-25 19:08:46,602 - WARNING - ec.trial_ok called before stimulus had stopped
2023-07-25 19:08:46,603 - EXP - Expyfun: Trial OK
2023-07-25 19:08:46,603 - INFO - Expyfun: Exiting
2023-07-25 19:08:46,609 - EXP - Expyfun: Audio stopped and reset.
Total running time of the script: ( 0 minutes 5.557 seconds)